本文描述了如何从零开始,发布一个自己编写NPM Module,并使用Git作为代码管理工具,对Module进行代码管理
下文的NPM用户名以npmuser代替,密码以xxx代替
Github用户名以gituser代替,密码以xxx代替
要发布的npm包名以module-name代替
1.注册NPM账号
既然要发布npm包,那么首先就需要注册npm账号,这样才能将发布npm包
2.建立Github仓库
所有的npm包实际上都需要定期更新,一是为了修改BUG,二是为了增加新的功能,这时候就需要用到版本控制,这里使用Git来管理代码,在Github上新建名为module-name的仓库,新建完成后,将仓库clone到本地
$ git clone git@github.com:gituser/module-name.git
3.修改npm仓库地址
首先明确一点,我们是要把我们的包发布到npm的官方网站,而不是镜像网站上,由于国内使用npm官方源安装Module时速度很慢,所以一般会使用淘宝的镜像,来加快速度,由于我们要发布npm包,这时候就需要修改源地址为官方源
$ npm config set registry https://registry.npmjs.org/
4.本地登陆npm账户
$ npm login
Username: npmuser
Password: xxx
Email: (this IS public) xxxxx@xx.com # 输入邮箱地址,绑定邮箱
npm notice Please check your email for a one-time password (OTP)
Enter one-time password: xxxxx # 会给邮箱阀验证码,这里输入验证码
Logged in as npmuser on https://registry.npmjs.org/.
当出现logged in as…就表明已经登陆成功了
5.初始化项目
由于npm已经发布了很多包,而npm要求每个包都不能重名,重名的包是无法发布的,所以这里为了防止冲突,引入scope,通过限定作用域来保证包的唯一性,这样就不用担心包名与其他人冲突。所谓作用域,就是给包名前面加上用户名来限定包,平时我们在安装npm包时,一般会遇到两种类型的包,一种是不带作用域的,如
$ npm install jest
另一种是带作用域的,如
$ npm install @zxkfall/audio-player
作用域的主要功能就是为了防止包名冲突,此外也会也会标明一类包,比如都是由zxkfall开发的包
初始化项目
$ cd module-name # 进入clone下来的代码仓库
$ npm init --scope=npmuser # 创建带有作用域的包
package name: (@npmuser/module-name) # 会直接采用文件夹的名字,也可以自己输入,注意作用域
version: (1.0.0) # 采用默认设置即可1.0.0
description: This is a module than can play music or other audio # 描述
entry point: (index.js) # 程序入口,默认index.js
test command: # 测试命令指定,暂时不指定,直接回车
git repository: https://github.com/gituser/module-name.git # 添加远程仓库地址
keywords: audio,musice,player
author: Xingkun Zhang
license: (ISC) Apache-2.0 # 指定开源协议为Apache-2.0
About to write to E:\study\project\module-name\package.json:
{
"name": "@npmuser/module-name",
"version": "1.0.0",
"description": "This is a module than can play music or other audio",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"repository": {
"type": "git",
"url": "git+https://github.com/gituser/module-name.git"
},
"keywords": [
"audio","music","player"
],
"author": "Xingkun Zhang",
"license": "Apache-2.0",
"bugs": {
"url": "https://github.com/gituser/module-name/issues"
},
"homepage": "https://github.com/gituser/module-namer#readme"
}
Is this OK? (yes) yes # 输入yes确认
之后便生成了package.json
文件
关于package.json
里面对应字段的含义可以在这里查看
实际上,我们初始化项目就是新建了一个package.json
文件,我们也可以自己新建一个package.json文件,根据对应字段的含义,自己定义内容
6. 安装@types/node
针对IDEA,有时候,IDEA会无法识别module关键字,这时候就需要安装
@types/node
,使module等关键字可用,不会被提示无法识别
$ npm install @types/node
7.添加index.js
文件
const createAudioPlayer = () => {
console.log('CreateAudioPlayer')
};
const AudioPlayer = function () {
console.log('new')
this.createAPlayer = createAudioPlayer
}
module.exports = AudioPlayer //要引出的模块名,其他项目如果要使用这个包,将会导入AudioPlayer
//由于定义了 this.createAPlayer = createAudioPlayer
//那么其他用户在使用时,就可以使用new AudioPlayer(.createAPlayer()这个函数
这是index.js
文件的一个基本模块,可用根据自己需要进行扩展
8.添加ignore
文件
对于git,我们需要添加.gitignore
文件来忽略一些文件的提交,内容如下
.idea/
node_modules/
package-lock.json
提交时将会忽略这些文件以及文件夹的内容
对于npm,我们期望包发布时,不要添加一些额外的文件,这里就需要用到.npmignore
文件,内容如下
test/
.idea/
node_modules/
.gitignore
package-lock.json
可以根据自己需要进行调整
此外还有一点需要注意的是,如果我们开发的包用到了第三方库,如果我们这个包没有这个库就无法运行,那么就不能作为测试库安装,否则就需要作为测试库进行安装。
eg
$ npm install axios # 如果需要用axios作为网络请求,这里就作为项目的依赖进行安装
$ npm install --save-dev jest # 由于jest仅仅用来在本地进行测试,所以应该作为测试库来安装
9.项目结构
module-name
- LICENSE
- index.js
- node_modules/
- package-lock.json
- package.json
- test/
10. 发布项目(不要着急发布)
$ npm publish --access=public
-
注意这里
--access=public
必须要加上,不然发布的是私有包,而NPM上要发布私有包是要收费的,如果第一步init时没有加上--scope=npmuser
,那么这里可以不加上这个后缀,因为不带作用域的包发布时,默认是公有的 - 发布项目后,72小时之后将无法删除项目,72小时之内,删除项目后,需要等待24小时才能发布同名的包
- 发布包后,此后每次发布包,都需要更改版本号,不然是无法再次发布的,这也很容易理解,你更新了项目,但是没有更新版本号,那用户又怎么知道你更新了内容。
11. 其他命令
修改仓库源为淘宝源
$ npm config set registry http://registry.npm.taobao.org/
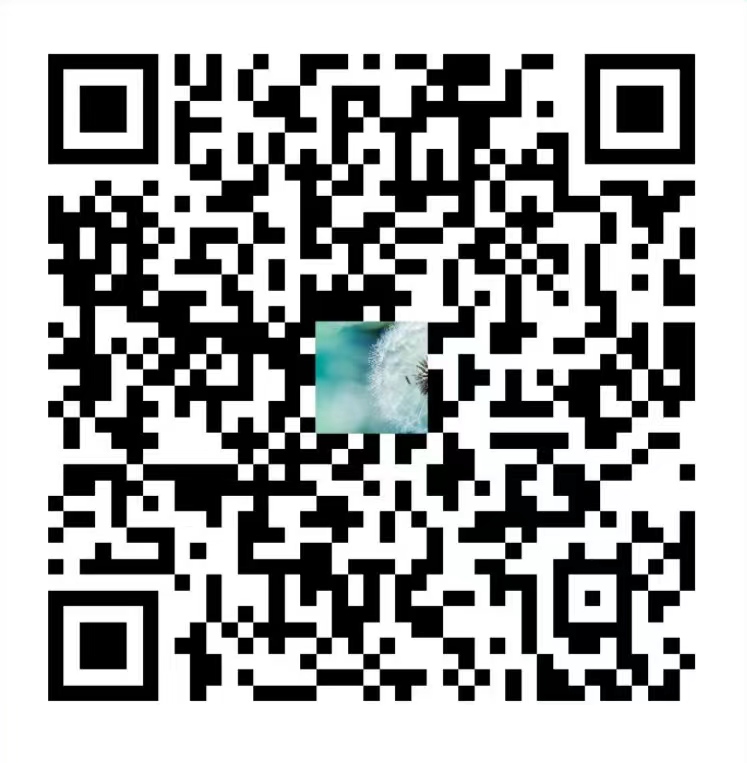